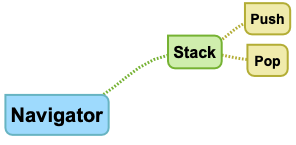
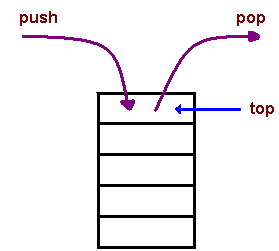
https://medium.com/@icelancer/flutter-navigator-1-basic-e300efb24543
flutter의 Navigator는 스택을 이용해 페이지를 관리할 때 사용하는 클래스이다.
- main.dart
import 'package:flutter/material.dart';
import 'package:subpage_example/page/first.dart';
import 'package:subpage_example/page/second.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
),
initialRoute: '/',
routes: {
'/': (context) => FirstPage(),
'/second': (context) => SecondPage(),
}
);
}
}
- page/first.dart
import 'package:flutter/material.dart';
import 'package:subpage_example/page/second.dart';
class FirstPage extends StatefulWidget {
@override
State<StatefulWidget> createState() => _FirstPageState();
}
class _FirstPageState extends State<FirstPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Sub Page Main'),
),
body: Container(
child: Center(
child: Text('첫 번째 페이지'),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
Navigator.of(context)
.pushNamed('/second');
},
child: Icon(Icons.add),
),
);
}
}
- page/second.dart
import 'package:flutter/material.dart';
class SecondPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Second Page'),
),
body: Container(
child: Center(
child: ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('돌아가기'),
),
),
),
);
}
}
Uploaded by Notion2Tistory v1.1.0